Mastering Java Collections for Efficient Data Handling
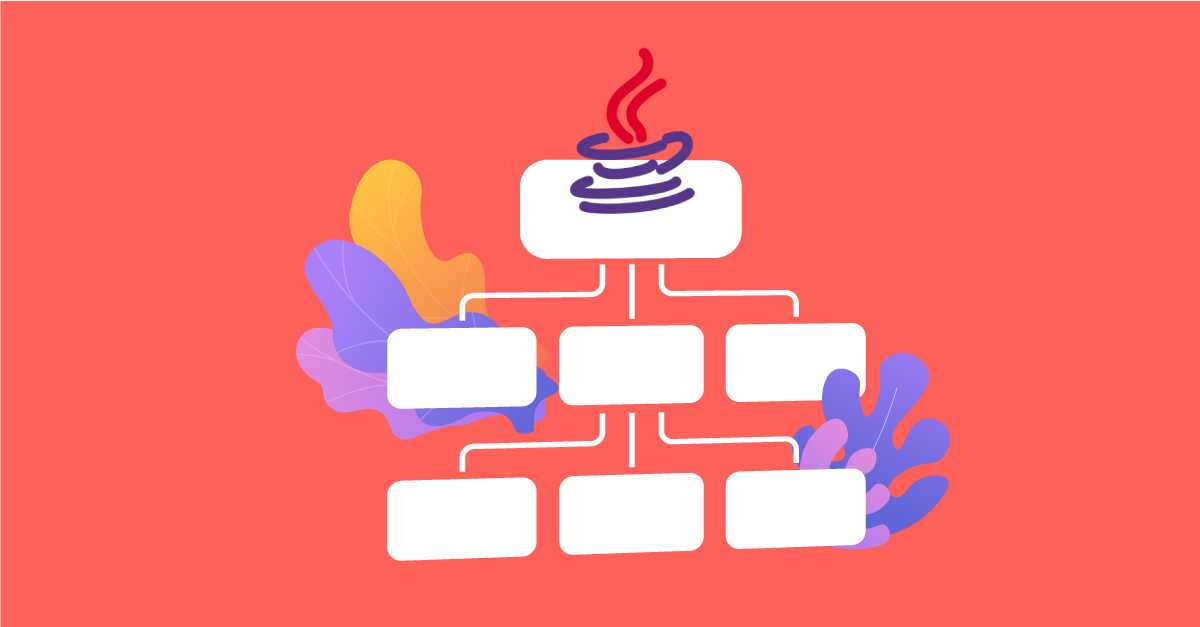
Introduction
One important and fundamental component of the Java programming language is the Java Collections Framework. It provides a comprehensive set of classes and interfaces to manipulate and store groups of objects. The Java Collections Framework aims to improve code reusability, boost expressiveness in Java programmes, and offer a standard architecture for describing and manipulating collections.
Java Collection Hierarchy
All of the interfaces and classes needed by the collection framework are included in the utility package java.utils. The Iterable interface, a feature of the collection framework, allows an iterator to cycle across every collection.
A collection in Java is a group of individual objects represented as a single unit by providing a number of classes and interfaces. It is the root interface of the collection framework. The primary child interfaces of the collection interface are List, Set, and Queue.
The Java Collection Framework also has the Map interface, however it does not inherit the Collection interface. When storing values as keys and value pairs, the map interface is the recommended choice.
The List interface in the Java Collections Framework represents an ordered collection of elements, where each element has an index or position. Lists allow for the storage of duplicate elements, and they maintain the order in which elements are inserted. This interface is a part of the broader collection hierarchy and extends the Collection interface.
Common implementations of the List interface include:
- ArrayList: Implements the List interface using a dynamic array, providing fast random access and efficient iteration.
- LinkedList: Implements the List interface using a doubly-linked list, allowing for efficient insertion and deletion operations.
- Vector: An older implementation that is synchronized, making it thread-safe. However, it is less efficient than ArrayList.
- Stack: Extends Vector and represents a last-in, first-out (LIFO) stack of objects.
ArrayList Implementation in Java
import java.util.ArrayList;
import java.util.List;
public class ArrayListExample {
public static void main(String[] args) {
// Creating an ArrayList of Strings
List<String> fruits = new ArrayList<>();
// Adding elements to the ArrayList
fruits.add("Apple");
fruits.add("Banana");
fruits.add("Orange");
// Accessing elements by index
System.out.println("First fruit: " + fruits.get(0));
// Iterating through the ArrayList
System.out.println("All fruits:");
for (String fruit : fruits) {
System.out.println(fruit);
}
// Checking if the ArrayList contains a specific element
String searchFruit = "Banana";
if (fruits.contains(searchFruit)) {
System.out.println(searchFruit + " is in the list.");
} else {
System.out.println(searchFruit + " is not in the list.");
}
// Removing an element from the ArrayList
String removedFruit = "Banana";
fruits.remove(removedFruit);
System.out.println("After removing " + removedFruit + ":");
for (String fruit : fruits) {
System.out.println(fruit);
}
// Checking the size of the ArrayList
System.out.println("Number of fruits in the list: " + fruits.size());
// Clearing all elements from the ArrayList
fruits.clear();
System.out.println("After clearing the list, size: " + fruits.size());
}
}
LinkedList Implementation in Java
import java.util.LinkedList;
public class LinkedListExample {
public static void main(String[] args) {
// Creating a LinkedList of Strings
LinkedList<String> countries = new LinkedList<>();
// Adding elements to the LinkedList
countries.add("USA");
countries.add("Canada");
countries.add("UK");
// Accessing elements by index
System.out.println("First country: " + countries.getFirst());
System.out.println("Last country: " + countries.getLast());
// Iterating through the LinkedList
System.out.println("All countries:");
for (String country : countries) {
System.out.println(country);
}
// Checking if the LinkedList contains a specific element
String searchCountry = "Canada";
if (countries.contains(searchCountry)) {
System.out.println(searchCountry + " is in the list.");
} else {
System.out.println(searchCountry + " is not in the list.");
}
// Removing an element from the LinkedList
String removedCountry = "Canada";
countries.remove(removedCountry);
System.out.println("After removing " + removedCountry + ":");
for (String country : countries) {
System.out.println(country);
}
// Checking the size of the LinkedList
System.out.println("Number of countries in the list: " + countries.size());
// Clearing all elements from the LinkedList
countries.clear();
System.out.println("After clearing the list, size: " + countries.size());
}
}
Stack Implementation in Java
import java.util.Stack;
public class StackExample {
public static void main(String[] args) {
// Creating a Stack of Strings
Stack<String> stack = new Stack<>();
// Pushing elements onto the stack
stack.push("Java");
stack.push("Python");
stack.push("C++");
// Printing the elements in the stack
System.out.println("Stack elements:");
for (String element : stack) {
System.out.println(element);
}
// Popping an element from the stack
String poppedElement = stack.pop();
System.out.println("Popped element: " + poppedElement);
// Printing the elements after popping
System.out.println("Stack elements after popping:");
for (String element : stack) {
System.out.println(element);
}
// Peeking at the top element without removing it
String topElement = stack.peek();
System.out.println("Top element without removing: " + topElement);
// Checking if the stack is empty
System.out.println("Is the stack empty? " + stack.isEmpty());
}
}
Queue Interface
A collection of elements sorted in a certain sequence, usually in accordance with the First-In-First-Out (FIFO) concept, is represented by the Queue interface in the Java Collections Framework. The order of elements is reversed (front/dequeue) and reversed (rear/enqueue). Queues are often used in scenarios where elements need to be processed in the order they were added.
Queue Implementation in Java
import java.util.LinkedList;
import java.util.Queue;
public class QueueExample {
public static void main(String[] args) {
// Creating a Queue of Strings using LinkedList
Queue<String> queue = new LinkedList<>();
// Enqueuing (adding) elements to the queue
queue.add("Task 1");
queue.offer("Task 2");
queue.add("Task 3");
// Dequeuing (removing) elements from the queue
String task1 = queue.remove();
System.out.println("Completed: " + task1);
String task2 = queue.poll();
System.out.println("Completed: " + task2);
// Peeking at the front element without removing it
String nextTask = queue.peek();
System.out.println("Next task: " + nextTask);
// Checking if the queue is empty
System.out.println("Is the queue empty? " + queue.isEmpty());
}
}
Set Interface
The Set interface extends the Collection interface and is part of the Java Collections Framework. It provides a collection of unique elements with no defined order. Sets are useful when you need to ensure that each element occurs only once in the collection. Common implementations of the Set interface include HashSet, TreeSet, and LinkedHashSet.
HashSet Implementation in Java
import java.util.HashSet;
import java.util.Set;
public class HashSetExample {
public static void main(String[] args) {
// Creating a HashSet of Strings
Set<String> uniqueWords = new HashSet<>();
// Adding elements to the HashSet
uniqueWords.add("Java");
uniqueWords.add("Python");
uniqueWords.add("C++");
// Adding a duplicate element (will not be added)
uniqueWords.add("Java");
// Printing the elements in the HashSet
System.out.println("Unique words:");
for (String word : uniqueWords) {
System.out.println(word);
}
// Checking if the HashSet contains a specific element
String searchWord = "Python";
if (uniqueWords.contains(searchWord)) {
System.out.println(searchWord + " is in the set.");
} else {
System.out.println(searchWord + " is not in the set.");
}
// Removing an element from the HashSet
String removedWord = "C++";
uniqueWords.remove(removedWord);
System.out.println("After removing " + removedWord + ":");
for (String word : uniqueWords) {
System.out.println(word);
}
// Checking the size of the HashSet
System.out.println("Number of unique words in the set: " + uniqueWords.size());
// Clearing all elements from the HashSet
uniqueWords.clear();
System.out.println("After clearing the set, size: " + uniqueWords.size());
}
}
Map Interface
The Map interface in the Java Collections Framework represents a collection of key-value pairs, where each key is associated with exactly one value. This interface provides a versatile way to store and retrieve data based on unique identifiers (keys). Common implementations of the Map interface include HashMap, TreeMap, and LinkedHashMap.
HashMap Implementation in Java
import java.util.HashMap;
import java.util.Map;
public class HashMapExample {
public static void main(String[] args) {
// Creating a HashMap with String keys and Integer values
Map<String, Integer> populationMap = new HashMap<>();
// Adding key-value pairs to the map
populationMap.put("USA", 331000000);
populationMap.put("China", 1444216107);
populationMap.put("India", 1393409038);
// Retrieving values based on keys
System.out.println("Population of China: " + populationMap.get("China"));
// Checking if a key exists in the map
String country = "India";
if (populationMap.containsKey(country)) {
System.out.println(country + " is in the map.");
} else {
System.out.println(country + " is not in the map.");
}
// Iterating through the map
System.out.println("All countries and their populations:");
for (Map.Entry<String, Integer> entry : populationMap.entrySet()) {
System.out.println("Country: " + entry.getKey() + ", Population: " + entry.getValue());
}
// Modifying the value associated with a key
populationMap.put("India", 1395000000);
System.out.println("Updated population of India: " + populationMap.get("India"));
// Checking the size of the map
System.out.println("Number of countries in the map: " + populationMap.size());
// Clearing all key-value pairs from the map
populationMap.clear();
System.out.println("After clearing the map, size: " + populationMap.size());
}
}
Differentiation of these interfaces and classes
ArrayList vs LinkedList
List interface vs Set interface
Set interface vs Map interface
Advantages of Java Collection Framework
- Ready-Made Tools: Java Collections Framework gives you a bunch of ready-made tools (classes and interfaces) to handle groups of things in your code.
- Save time: You don’t have to build basic data structures from scratch. Just use what Java gives you, saving you time and effort.
- Better Code Quality: Because these tools are well-built, your code can be more reliable and efficient.
- Maintenance: It is simple to maintain code created with the aid of the Java Collections framework because the majority of the framework’s code is open source and there are many API documents available.